Labwork 5¶
Problem 1: Edge Tracking¶
An often applied procedure in image segmentation is edge tracking, in which the outline of objects is reconstructed after edge detection. In this assignment, you are going to study a simplified edge tracking algorithm which is described in the lecture notes. In the literature this technique is often referred to as “Dynamic programming”. Briefly, you need to implement these steps:
- Calculate a cost matrix C(r,c) in which r and c are the rows and the columns of an image f (
% TODO 1
):- C(r,c) = 1 - \sqrt{f_x^2(r,c)+f_y^2(r,c)}/\max\limits_{\forall{r,c}}(\sqrt{f_x^2(r,c)+f_y^2(r,c)}) in which f_x and f_y are the directional derivatives of the image along x and y.
-
Compute a cumulative cost matrix A(r,c) (
% TODO 2-4
). Here, A(0,c)=0 andA(r,c)=C(r,c)+\text{min}\{A(r-1,c-1),A(r-1,c),A(r-1,c+1)\}. -
Select the minimum value on the last row of A and trace back the minimal cost path along A. The projection of the minimum cost path on C is your final segmentation.
Hint
You can compute the cost matrix C(r,c) entirely in vectorial form without a for
loop. Remember the element-wise multiplication operator from Labwork 3. There are similar operators for division and exponentiation in MATLAB.
A few synthetic toy images as shown in Figure 1 are available to you in script.m
. Implement the edge tracking routine and visualize the detected edge on top of these images. Explain what is happening and of course why!

Problem 2: Mean shift algorithm¶
A region growing-based segmentation algorithm that was presented in the lectures is the mean shift algorithm. In this assignment, you are going to use this algorithm to segment objects from an image based on the color information.
We have provided you with almost completed code to do so, see script.m
. You only need to complete the function MoveMean()
which updates the mean of the data points within a neighbourhood and checks if this mean value has reached convergence or not (% TODO 5-8
). Please have a look at script.m
to see how the color information is mapped to data points and then complete the function. Figure 2 shows an example outcome of such a function.
The mean shift algorithm has only one free parameter (radius
) which defines the search window size. Try different values for this parameter and explain its effects on the segmentation result. Keep the free parameter constant and try to smooth the input image with different degrees of smoothing before starting the segmentation. Compare the result of these experiments.
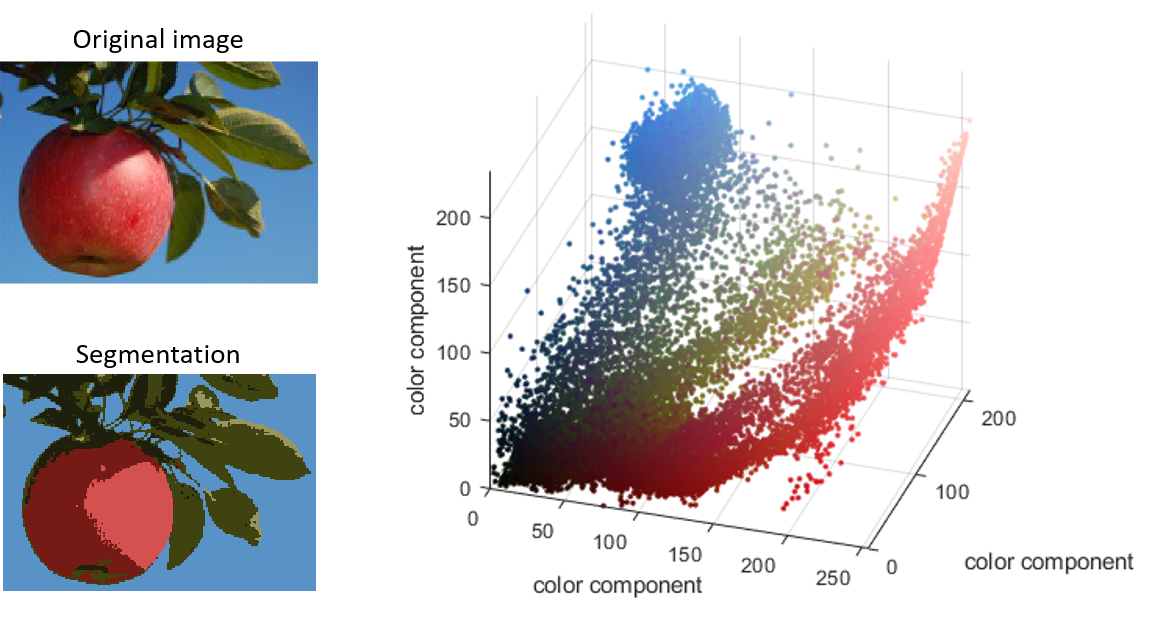
Problem 3: Template matching¶
In this assignment, you are going to implement a template matching algorithm for segmenting a region of interest (ROI) inside an image. Open script.m
and follow the steps to reproduce the example template matching problem that is shown in the lecture notes.
Your first task is to find the right eye of erika.tif
using eye.ics
as the template (Figure 3). Plot the heatmap which visualizes the correlation score of each pixel while the template slides over the entire image (% TODO 9-11
). Explain why certain regions yield a high response. How is this related to the template? Is the response around the correct position symmetrical?
In certain applications, there might be more than one single instance of the object of interest present in the image. Therefore, it is desirable to develop algorithms which are able to identify all objects that are similar to a template in an image.

Optional
Multiple instances of the template
Your task (% TODO 12-13
) is to extend the routine to detect instances of the nuclear pore complexes (NPCs) in the whole field of view of a superresolution microscope (Figure 4). The NPC is a cell structure which geometrically can be described as an eight-fold symmetric ring. You are already provided with a template (“npc_template.tiff”) but you can also choose your own template by selecting and cropping a different NPC from “npc_fov.tiff”. How many NPCs can you detect? Try to find a way to discard false positive detections (detected ROIs which are not NPC). Try different templates and report how the number of matches varies?
!
Files¶
You need to download the following scripts and images for this labwork.